C and C programming languages provide rand and srand functions in order to create random numbers. Random numbers can be used for security, lottery etc. Random numbers can be used for security, lottery etc. Srand(time(NULL)) in C duplicate This question already has an answer here: If I comment out the line with srand, the program will work, but there is no seed so the values will be the same each time. The assignment requires that I use rand, srand, and time to have the dice function be completely random.
C program to generate pseudo-random numbers using rand and random function (Turbo C compiler only). As the random numbers are generated by an algorithm used in a function they are pseudo-random, this is the reason that word pseudo is used. Function rand() returns a pseudo-random number between 0 and RAND_MAX. RAND_MAX is a constant which is platform dependent and equals the maximum value returned by rand function.
C programming code using rand
We use modulus operator in our program. If you evaluate a % b where a and b are integers then result will always be less than b for any set of values of a and b. For example
For a = 1243 and b = 100
a % b = 1243 % 100 = 43
For a = 99 and b = 100
a % b = 99 % 100 = 99
For a = 1000 and b = 100
a % b = 1000 % 100 = 0
In our program we print pseudo random numbers in range [0, 100]. So we calculate rand() % 100 which will return a number in [0, 99] so we add 1 to get the desired range.
#include <stdio.h>#include <stdlib.h>
int main(){
int c, n;
printf('Ten random numbers in [1,100]n');
for(c =1; c <=10; c++){
n =rand()%100+1;
printf('%dn', n);
}
return0;
}
If you rerun this program, you will get the same set of numbers. To get different numbers every time you can use: srand(unsigned int seed) function; here seed is an unsigned integer. So you will need a different value of seed every time you run the program for that you can use current time which will always be different so you will get a different set of numbers. By default, seed = 1 if you do not use srand function.
C programming code using random function (Turbo C compiler only)
Function randomize is used to initialize random number generator. If you don't use it, then you will get same random numbers each time you run the program.
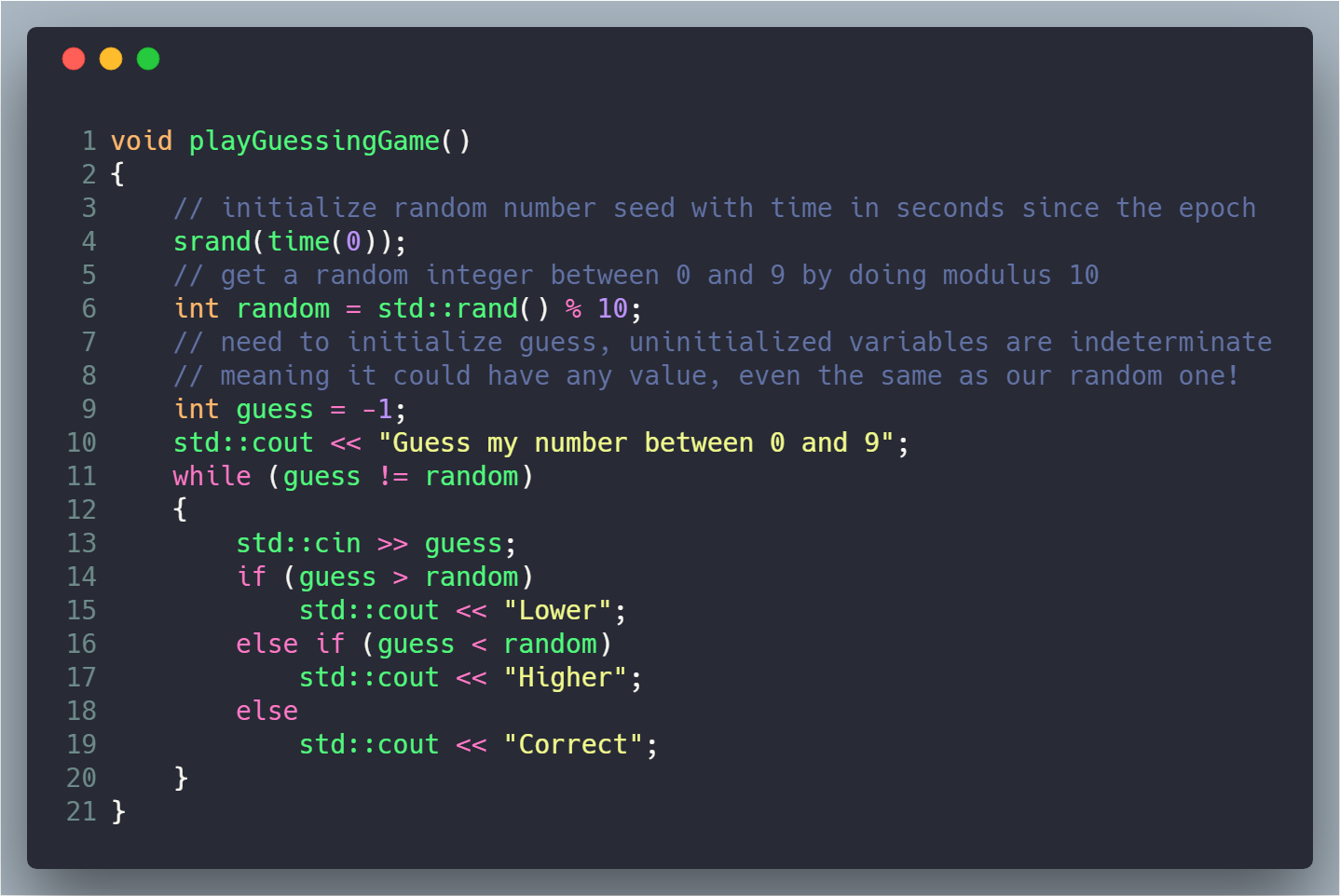
#include <conio.h>
How To Use Rand In Dev C 5
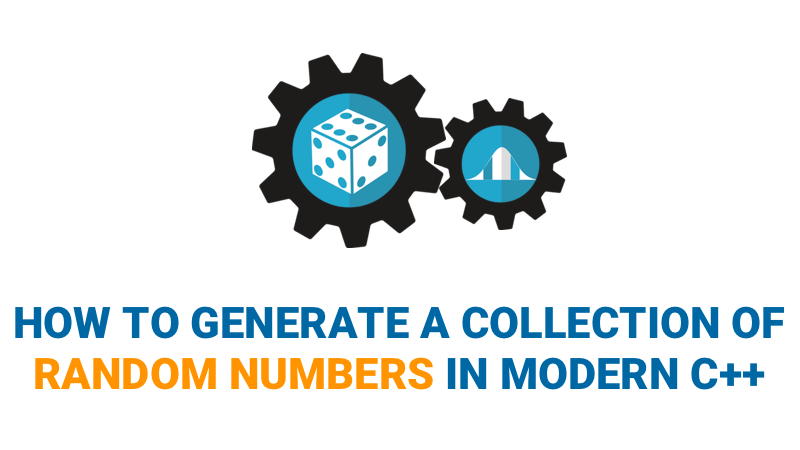
#include <stdlib.h>
int main()
{
int n, max, num, c;
printf('Enter the number of random numbers you wantn');
scanf('%d',&n);
printf('Enter the maximum value of random numbern');
scanf('%d',&max);
printf('%d random numbers from 0 to %d are:n', n, max);
randomize();

for(c =1; c <= n; c++)
{
num = random(max);
printf('%dn',num);
}
getch();
return0;
}
How To Use Rand In Dev C Download
The main problem for distribution is that people don't know how to properly get a random number from rand() -- there is no magic library code to do it for you -- and most people get it Wrong.
The next is that rand's range is often significantly smaller than you want it to be.
You can read all about rand() on it's FAQ:
http://www.cplusplus.com/faq/beginners/random-numbers/
If you want to get technical, rand() implementations tend to favor lower bits, which leads to biased numbers.
The C++ <random> library gives you much more powerful PRNGs with a lot of built-in ways to draw numbers from them without bias.
The most difficult thing to do is seed it properly. Unfortunately, people still depend on the clock -- which is a mistake, but acceptable for video games and the like -- anything that doesn't require statistical correctness (like Monte Carlo simulations and the like).
You need to warm up your Mersenne Twister.
generator.discard(10000)
or so.Hope this helps.