Jan 30, 2013 int main (int argc, char.argv) Here, argc parameter is the count of total command line arguments passed to executable on execution (including name of executable as first argument). Argv parameter is the array of character string of each command line argument passed to executable on execution. To use command line arguments in your program, you must first understand the full declaration of the main function, which previously has accepted no arguments. In fact, main can actually accept two arguments: one argument is number of command line arguments, and the other argument is a full list of all of the command line arguments.
In Unix, when you pass additional arguments to a command, those commands must be passedto the executing process. For example, in calling ls -al, it executes the programls and passes the string -al as an argument:
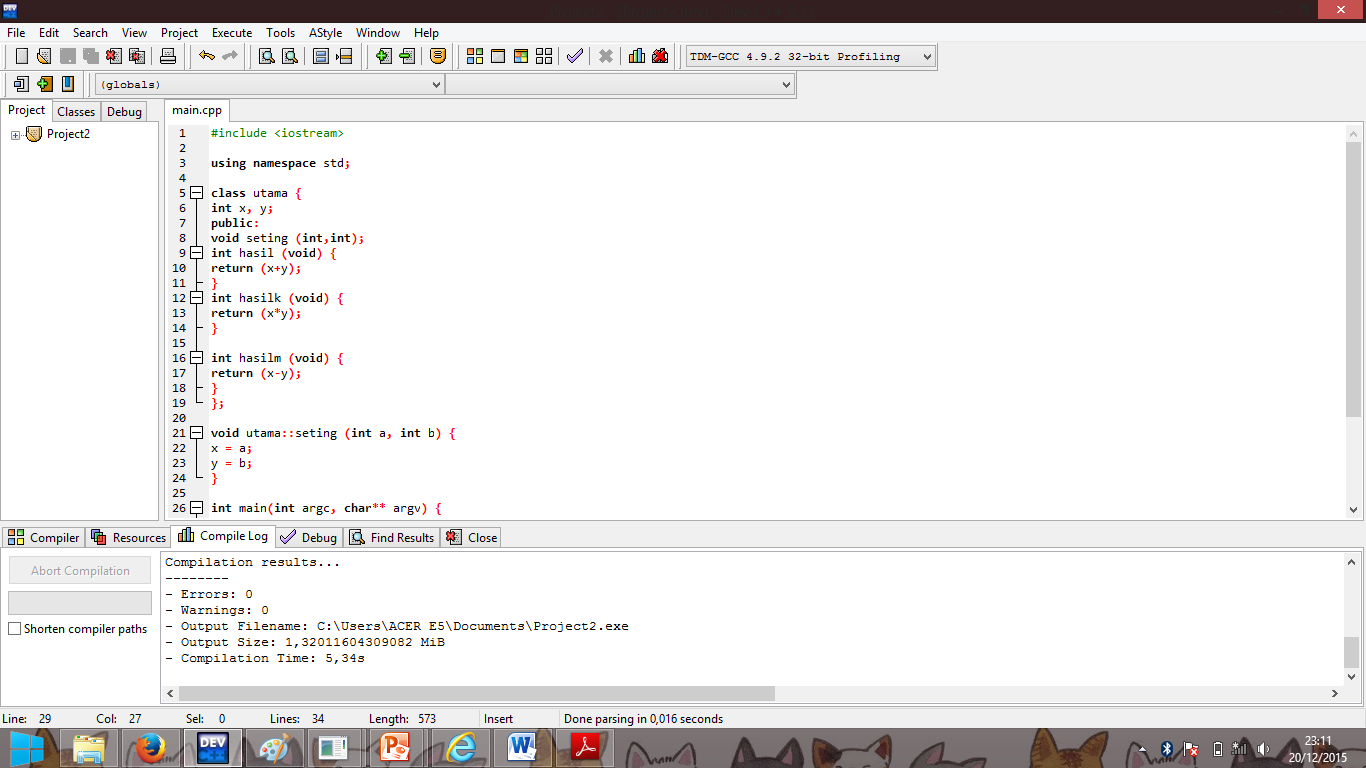
The way a running program accesses these additional parameters isthat these are passed as parameters to the function main:
Here argc means argument count andargument vector.
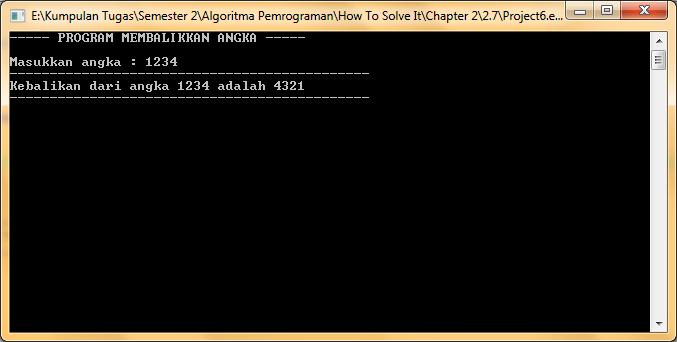
The first argument is the number of parameters passed plus one to include thename of the program that was executed to get those process running. Thus,argc is always greater than zero and argv[0] is the name ofthe executable (including the path) that was run to begin this process. For example, if we run
Here we compile this code and first compile and run it so that the executable name is a.out and thenwe compile it again and run it so the executable name is arg:
If more additional command-line arguments are passed, the string of all characters is parsedand separated into substrings based on a few rules; however, if all the characters are eithercharacters, numbers or spaces, the shell will separate the based on spaces and assign args[1]the address of the first, args[2] the address of the second, and so on.
The following program prints all the arguments:
Here we execute this program with one and then twelve command-line arguments:
For Fun
Now, you may be wondering what actually happens (and if you don't, you're welcome to skip this).First, when the command is executed, the shellparses the command line and separates the individual pieces by a null character 0. For example,the execution of
has the shell generate the string ./a.out☐first☐second☐third☐fourth☐fifth☐where the box represents the null character. Next, memory for an array of six (argc) pointers is allocatedand these six pointers are assigned the first character of each of the strings into which the command line was parsed.Finally, memory is allocated for the stack and using 8-byte alignment, the two arguments are placed into the stack.This is shown in Figure 1.
Figure 1. The result of calling ./a.out first second third fourth fifth on the command line.
Further Fun
In Unix, the shell does further processing of the command. For example, if it finds wild cards that indicate filesin the current directory, it will attempt to expand those wild cards. These include ? for one unknowncharacter and * for any number of characters.
For example, the following examines the file in the current directory:
Similarly, you can tell the command line to treat spaces as part of one string or ignore wildcardsby either using the backslash before thespace or wildcard or surrounding the string by single or double quotes.
Finally, if you surround text with backticks, it will execute that first and then replace that withthe output of the command:
Argc Argv Dev C File
Guess what happens if you enter ./a.out `ls -al`.